Create a Flappy Bird Clone With Python P4
Posted in tutorials python -This is one part of a multi-part tutorial. To see other posts in the same series, please click below:
Part 4 - Make a “flapping” flappy bird
Part 4: Make a “flapping” flappy bird
Now that the bird is loaded and floating on our black screen, the next thing we would want to do is to load the background image, and at the same time, set the screen size to the size of the background. These can be done with the function get_size
we have figured out last time.
First we need to load the background image. There are two of them in the images
directory, of which one is called background-day
and the other background-night
. You can choose either, but I like day better, so I will pick background-day
.
background = pygame.image.load("images/background-day.png")
The size
variable, which is used in pygame.display.set_mode(size)
, is now hard-coded. We, instead, want to set it to whatever the size of the background image is:
size = width, height = background.get_size()
Now, let’s use blit
to draw the background image on top of the screen. This can be done outside the loop, but remember, when we add the moving stuff (the tubes) into the game, we will have to re-draw the background in every iteration, hence putting it inside the loop makes more sense. However, the background must be blit
before everything else, so that everything else is drawn on top of the background, not the other way around.
while 1:
for event in pygame.event.get():
if event.type == pygame.QUIT: sys.exit()
screen.blit(background, (0, 0))
screen.blit(bird, (0, bird_y_pos))
VoilĂ , we’ve got a bird and a background.
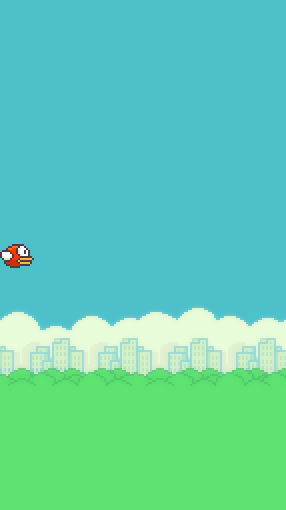
The next thing we want to do is to make the bird flapping its wings. As we’ve talked about, a “movement effect” can be achieved by making an image change in every iteration, which “tricks” our brains that the object is moving. That’s why we have upflap
, midflap
and downflap
images for the same bird. So if you change from upflap
to midflap
in the first frame, midflap
to downflap
in the next frame, then downflap
to midflap
afterwards, etc. you can make it look like the same bird image is flapping (while it’s actually three images).
But how exactly do we do it? We can create a list (or a tuple) of bird images, and simply loop through it back and forth. Let’s start with importing all the images and putting them into a list
bird_upflap = pygame.image.load("images/redbird-upflap.png")
bird_midflap = pygame.image.load("images/redbird-midflap.png")
bird_downflap = pygame.image.load("images/redbird-downflap.png")
bird_images = [bird_upflap, bird_midflap, bird_downflap]
As we now remove the initial bird
variable, we have to use one of these images to determine the bird_height
instead. You can use any of them (after all, they are supposed to represent the same bird).
Now let’s get to the logic that makes the bird flap. Outside the loop, we set the following variables:
bird_idx = 0
increment = 1
And inside the loop, we can access different bird image like this:
# Determine the current bird
bird = bird_images[bird_idx]
bird_idx += increment
# Change increment direction if necessary
if bird_idx >= 2 or bird_idx <= 0:
increment = -increment
What happens there is we set the initial bird to be the first bird on our list, then in every iteration, we increase the bird_idx
by increment
(i.e. 1) (so that we get the next bird on the list in the list in next iteration). When the bird_idx
reaches 2
, which means we cannot increase it anymore, we change the direction of the increment
, so that next time the bird_idx
changes, it decreases by 1 instead. The increment
changes again to 1
when the bird_idx
is 0
and so on.
What we achived is this nice flapping bird:
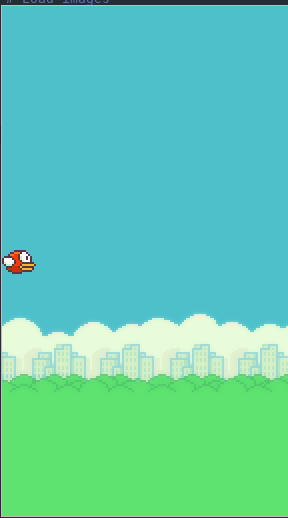
Our code now looks like this (I made some arrangement so it looks better, but whether or not you do it shouldn’t matter):
import sys, pygame
pygame.init()
# Load images
background = pygame.image.load("images/background-day.png")
bird_upflap = pygame.image.load("images/redbird-upflap.png")
bird_midflap = pygame.image.load("images/redbird-midflap.png")
bird_downflap = pygame.image.load("images/redbird-downflap.png")
bird_images = [bird_upflap, bird_midflap, bird_downflap]
size = width, height = background.get_size()
screen = pygame.display.set_mode(size)
bird_height = bird_upflap.get_height()
bird_y_pos = int(height/2 - bird_height/2)
bird_idx = 0
increment = 1
while 1:
for event in pygame.event.get():
if event.type == pygame.QUIT: sys.exit()
# Determine the current bird
bird = bird_images[bird_idx]
bird_idx += increment
# Change increment direction if necessary
if bird_idx >= 2 or bird_idx <= 0:
increment = -increment
screen.blit(background, (0, 0))
screen.blit(bird, (0, bird_y_pos))
pygame.display.flip()
As the bird is now flapping, let’s make it “fly” next time. See you then!
To see other posts in the same series, please click below: